반응형
- 객체의 기능을 구현하기 위해 클래스 내부에 구현되는 함수
- 멤버 함수이라고도 함
- 메서드를 구현함으로써 객체의 기능이 구현 됨
- 메서드의 이름은 그 객체를 사용하는 객체에 맞게 짓는것이 좋음
내부 클래스 함수 이용 예제
public class FuntionTest {
public static void main(String[] args) {
int n1 = 10;
int n2 = 20;
int total = addNum(n1, n2);
System.out.println(total);
sayHello("안녕하세요");
total = calcSum();
System.out.println(total);
}
public static int addNum(int num1, int num2) {
int result;
result = num1 + num2;
return result;
}
public static void sayHello(String greeting) {
System.out.println(greeting);
}
public static int calcSum() {
int sum = 0;
int i;
for (i = 0; i< 100; i++) {
sum += i;
}
return sum;
}
}
결과
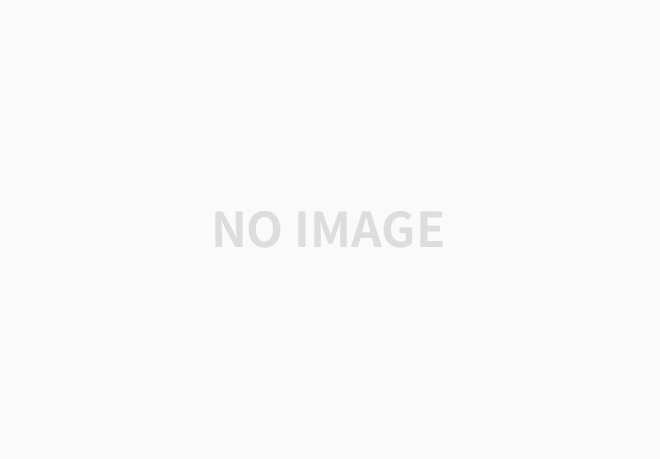
외부클래스 함수를 가져오는 예제
데이터를 저장 시킬 클래스
public class Student {
public int studentID;
public String studentName;
public String address;
public void showStudentInfo() {
System.out.println(studentID + "학번 학생의 이름은 " + studentName + " 이고, 주소는 " + address + "입니다.");
}
public String getStudentName() {
return studentName;
}
public void setStudentName(String name) {
studentName = name;
}
}
실행 시킬 메인 클래스
package ch04;
public class StudentTest {
public static void main(String[] args) {
Student studentLee = new Student();
studentLee.studentID = 12345;
studentLee.setStudentName("Lee");
studentLee.address = "서울 강남구";
studentLee.showStudentInfo();
Student studentKim = new Student();
studentKim.studentID = 54321;
studentKim.setStudentName("Kim");
studentKim.address = "서울 강서구";
studentKim.showStudentInfo();
}
}
결과
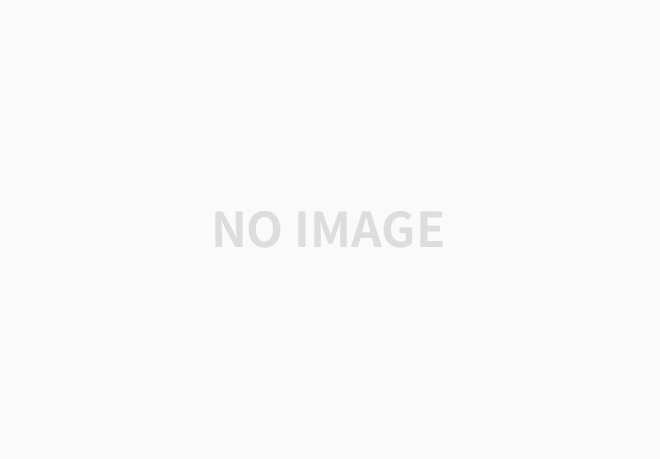
반응형
'언어 > JAVA' 카테고리의 다른 글
생성자 (0) | 2021.04.12 |
---|---|
인스턴스, 힙 메모리, 용어 정리 (0) | 2021.04.12 |
객체 지향 프로그램 (0) | 2021.04.12 |
중간에 멈추는 break문, 무시하고 계속 진행하는 continue문 (0) | 2021.04.11 |
반복문 (0) | 2021.04.11 |